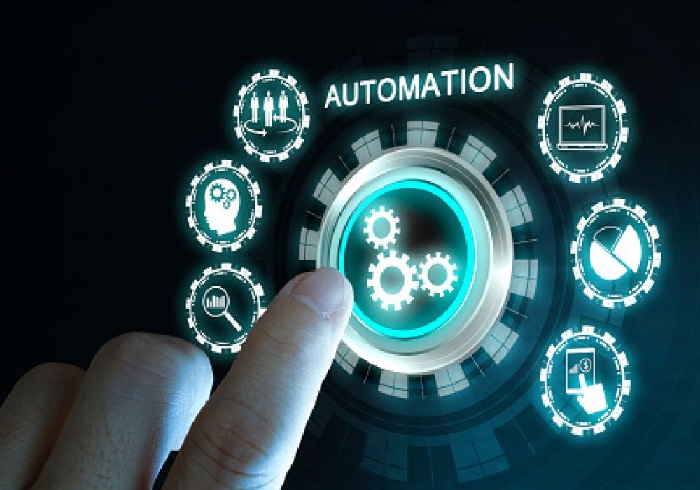
How To Run Your Regression Automation Testing Scripts With Selenium?
In software development, ensuring the functionality and reliability of your application is paramount. One crucial component to maintain this standard is through regression testing. Essentially, regression testing confirms that new changes in the code do not adversely affect the existing functionalities. It’s a repetitive but vital task, often requiring a rerun of numerous test cases. Manual testing can be cumbersome and prone to errors, which is why automation is gaining traction.
Using Selenium, a powerful tool for controlling a web browser through programs, you can create scripts to automate your regression tests, making them more efficient, accurate, and cost-effective. In this article, we will delve into how you can run your regression automation testing scripts with Selenium, streamlining your testing process and ensuring that your application performs optimally at all times.
Step-By-Step Guide To Run Your Regression Automation Testing Scripts With Selenium
Running regression automation testing scripts with Selenium involves a series of steps to ensure that your web application functions as expected and remains stable after each release. Here’s a guide to help you get started:
Set Up Your Environment
Start by installing the necessary tools for automation testing. Install the Java Development Kit (JDK), an Integrated Development Environment (IDE) like Eclipse or IntelliJ, Selenium WebDriver, and a build tool like Maven or Gradle for dependency management.
Create Your Automation Project
Create a new Java project in your chosen IDE. Add the Selenium WebDriver library to your project. If you’re using Maven, add the Selenium dependency to your `pom.xml` file:
“`xml
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>3.141.59</version>
</dependency>
</dependencies>
“`
Design Your Test Cases And Write Automation Scripts
Identify the test scenarios you want to automate, such as user login, form submission, or navigation. Break down these scenarios into smaller steps that Selenium can perform. Then create Java classes to represent your test cases. Implement the Page Object Model (POM) design pattern to organize your code. For example, if you have a login page, create a corresponding `LoginPage` class with methods to interact with page elements:
“`java
public class LoginPage {
private WebDriver driver;
public LoginPage(WebDriver driver) {
this.driver = driver;
}
public void enterUsername(String username) {
WebElement usernameField = driver.findElement(By.id(“username”));
usernameField.sendKeys(username);
}
public void enterPassword(String password) {
WebElement passwordField = driver.findElement(By.id(“password”));
passwordField.sendKeys(password);
}
public void clickLoginButton() {
WebElement loginButton = driver.findElement(By.id(“loginButton”));
loginButton.click();
}
}
“`
Set Up Test Data And Implement Assertions
Prepare test data for your test scenarios. This could involve creating mock user accounts or generating sample input data for forms. Next, use testing frameworks like JUnit or TestNG for assertions. After each action, add assertions to validate expected outcomes. For example, with JUnit:
“`java
import org.junit.Assert;
import org.junit.Test;
public class LoginTest {
@Test
public void testValidLogin() {
WebDriver driver = new ChromeDriver(); // Initialize driver
LoginPage loginPage = new LoginPage(driver);
loginPage.enterUsername(“testuser”);
loginPage.enterPassword(“password”);
loginPage.clickLoginButton();
// Assuming successful login redirects to dashboard
String expectedUrl = “https://example.com/dashboard”;
String actualUrl = driver.getCurrentUrl();
Assert.assertEquals(expectedUrl, actualUrl);
driver.quit();
}
}
“`
Configure Test Run
Configuring your test run is an essential step in automated web testing, ensuring that everything runs as expected. Firstly, you’ll need to specify the WebDriver configuration. Once you’ve configured WebDriver, you’ll often want to specify other configurations for your test run. For example, you can set test timeouts, specify test groups, order test execution, and so forth. Both JUnit and TestNG offer rich sets of annotations and configuration options to tailor the test execution to your needs.
Test frameworks like TestNG and JUnit also provide ways to parameterize your tests, allowing you to run the same test multiple times with different data sets. This is incredibly useful for covering various test scenarios. After setting all configurations, you’re ready to run your tests.
Run Your Test Scripts
Execute your test scripts using the testing framework’s run command. This will trigger the WebDriver to interact with the web pages and perform the actions specified in your scripts. Once WebDriver is initialized, it will start executing the test scripts line by line. These scripts will open a web browser window, navigate to specified URLs, and interact with web page elements, all according to the commands you’ve written in your test cases. This can include a wide range of actions such as clicking buttons, filling out forms, or even executing JavaScript code within the web page. The WebDriver behaves as if a real user is interacting with the web application, but it does so in an automated and controlled manner.
As the test scripts run, they also usually contain assertions or checkpoints to verify that certain conditions are met—like confirming that clicking a button takes you to the correct page or that an error message appears when it should. These assertions help in determining whether the test case has passed or failed.
Review Test Results
Once all test cases have been executed, you must go through the results generated by the testing framework meticulously. This involves identifying whether each test passed or failed, and if it failed, understanding why that happened. Often, the framework will provide detailed logs, error messages, and sometimes screenshots to assist in diagnosing the issue. These resources are invaluable for debugging, as they offer clues and insights into what went wrong during the test execution. At this point, you’ll need to modify your test script or perhaps even the application code itself to resolve the issue. Make the necessary changes cautiously, ensuring you’re not introducing new issues in the process.
Once the modifications are complete, rerun the test to validate that the issue has been adequately addressed. If the test passes, you can proceed with confidence, but be prepared to cycle back through this debugging process if subsequent tests reveal additional failures or issues.
Maintain Test Scripts
Maintaining test scripts is an ongoing necessity in software development, particularly in environments where applications are frequently updated or changed. As your application evolves—be it changes in the user interface, updates in functionality, or new features—your test scripts must be updated to match this evolving landscape. If not, they risk becoming obsolete, failing to catch crucial bugs or flaws that could impact the user experience. For example, if a button’s ID changes in the UI, a Selenium script designed to click that button will fail unless updated with the new ID.
Similarly, test data needs to be kept up-to-date to reflect real-world scenarios that the application might encounter. The initial conditions and data sets that were valid at the beginning of the project may no longer be representative, which can affect the accuracy and relevance of your testing results. Moreover, the assertions—the conditions or boolean expressions that the software should satisfy—must also be revised. If a new feature is added or if existing functionality is modified, the original assertions may no longer hold true or cover all the necessary ground. You might need to add new assertions or modify existing ones to verify that the updated features are working as expected.
Automate Test Execution
Automating test execution through a continuous integration (CI) pipeline is an invaluable strategy for achieving quicker and more reliable software delivery. Tools like Jenkins or GitLab CI/CD provide an automated way to build, test, and deploy code changes in real time, offering immediate feedback to the development team. This is particularly advantageous for regression testing—a type of testing aimed at ensuring that new changes haven’t adversely affected the existing functionalities of the application. Automating regression tests within a CI pipeline means that these tests can be executed automatically every time a code change is committed to the repository.
In a typical setup, a developer commits code to a version control system like Git. This triggers the CI pipeline, which may start by building the application and running unit tests. Once those are successful, the pipeline can then execute a suite of automated regression tests designed to validate existing functionalities. Because this occurs after each commit, the team receives instant feedback and can immediately address any issues, such as broken code or failed tests. This significantly reduces the time between identifying and fixing errors, leading to faster development cycles and a more stable application.
Best Practices For Writing Efficient And Maintainable Regression Automation Scripts
Writing efficient and maintainable regression automation scripts is crucial for long-term success and a smooth testing process. Here are some best practices to follow:
Modularize Test Code
Modularizing test code involves breaking down your script into smaller, reusable functions and modules. This helps in reusing the same piece of code across multiple test scripts, making it efficient and easier to maintain. Any changes in the application’s behaviour can be reflected by making updates to a single module instead of updating every test script that contains that particular piece of logic.
Include Exception Handling
Robust scripts include appropriate exception-handling mechanisms. These ensure that your test execution doesn’t halt unexpectedly but instead logs an error message and moves on to the next test case. Exception handling contributes to both efficiency and maintainability by making it easier to troubleshoot issues as they arise.
Avoid Hardcoded Values (Magic Numbers)
Hardcoded values or ‘magic numbers’ can make your script brittle and difficult to maintain. Instead, use constants or externalize these values in configuration files. This way, if there’s a need to update any value, you can do it in one place without hunting through the entire codebase.
Implement Test Retries For Flaky Tests
Although it’s essential to address the root causes of flaky tests, sometimes a temporary solution like implementing test retries can be helpful. This means if a test fails, it’s retried once or twice more before being marked as failed. This can handle sporadic issues, but always ensure you eventually address the root cause of the flakiness.
Conclusion
Automating your regression tests with Selenium not only saves valuable time but also increases the efficiency and accuracy of your testing cycle. The power of Selenium to script complex scenarios across multiple browsers and operating systems makes it an indispensable tool in any tester’s kit. However, the combination of Selenium with LambdaTest takes things a notch higher by providing a cloud-based environment that facilitates cross-browser testing over 3000+ different browser and operating system combinations. This dynamic duo ensures that you deliver a bug-free and high-quality product to the market at a much faster pace.
By leveraging Selenium for scripting and LambdaTest for a scalable, reliable testing environment, you’re arming yourself with a robust framework that can tackle the most demanding of regression tests.