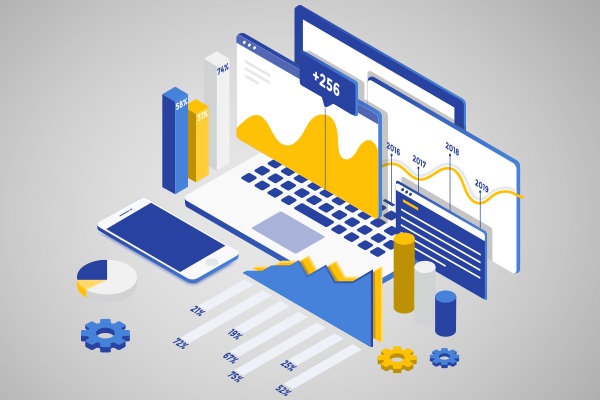
How To Run Your Cucumber Automation Testing Scripts With Appium?
Today ensuring the quality and reliability of mobile applications is more critical than ever. This is where Appium automation comes into play, offering a seamless way to conduct automated tests on various mobile platforms. Further, combining Appium with Cucumber expands your testing capabilities, allowing you to write human-readable descriptions of software behaviours without detailing how that functionality is implemented. When it comes to running these tests in a scalable and reliable manner, LambdaTest serves as a robust cloud-based platform that enables you to perform automated cross-browser testing across 3000 browsers, devices, and operating systems, making it a top choice among other cloud testing platforms. .
In this article, we will explore the steps to run your Cucumber automation testing scripts with Appium. So, let’s start right away without any more waiting…
Steps To Run Your Cucumber Automation Testing Scripts With Appium
Below are the instructions to set up and run your Cucumber automation testing scripts with Appium:
Install Required Software
Before setting up your Cucumber automation testing scripts with Appium, ensure you have the necessary software installed. Start by installing the Java Development Kit (JDK) of version 8 or above. Additionally, you’ll need to set up Maven, a build automation tool for Java projects, to manage dependencies and build your project. Install Appium using Node.js and npm (Node Package Manager), which allows you to interact with the Appium server and automate mobile applications. Finally, choose an Integrated Development Environment (IDE) such as IntelliJ IDEA or Eclipse to write, organize, and manage your code effectively.
Create A New Maven Project
Begin by creating a new Maven project in your chosen IDE. A Maven project provides a structured foundation for your automation tests. In the project’s `pom.xml` file, you’ll define essential project information, including dependencies for both Cucumber and Appium libraries. These dependencies ensure that your project has access to the required classes and methods to work with Cucumber and Appium seamlessly.
<dependencies>
<!– Cucumber dependencies –>
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-java</artifactId>
<version>6.10.4</version>
</dependency>
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-junit</artifactId>
<version>6.10.4</version>
<scope>test</scope>
</dependency>
<!– Appium Java Client –>
<dependency>
<groupId>io.appium</groupId>
<artifactId>java-client</artifactId>
<version>7.5.1</version>
</dependency>
<!– Other dependencies as needed –>
</dependencies>
Project Structure
Organize your project’s directory structure for clarity and maintainability. Typically, the project structure involves a `src` folder with `main` and `test` subdirectories. Under `main`, you’ll find `java` and `resources` directories, while under `test`, you’ll have `java` and `resources` subdirectories as well. The `java` folders will hold your source code, including step definitions, while the `resources` folders will contain configuration files and other resources needed for testing. Within the `resources` directory, create subfolders like `features` to store your Gherkin feature files and `app` to store the mobile application file.
Create Feature Files
Craft your test scenarios using Gherkin syntax in `.feature` files. These files, located in the `features` directory, provide a human-readable representation of test scenarios. Each scenario consists of steps written in plain language. These steps will be mapped to Java methods in your step definition classes. Feature files serve as the bridge between non-technical stakeholders and the automated tests, making it easier to understand what the tests are intended to accomplish.
For example, ‘login.feature’:
Feature: User Login
Scenario: Valid user can log in
Given the user is on the login screen
When they enter valid credentials
Then they should be logged in
Write Step Definitions
Develop the step definition classes in the `stepDefinitions` package within the `test/java` directory. These classes contain Java code that corresponds to the Gherkin steps outlined in your feature files. You’ll use the Appium APIs to interact with the mobile application. Appium provides methods to locate elements, perform actions, and verify the behaviour of your application during testing. By writing these step definitions, you’re essentially creating the automation logic that drives your tests. For example, ‘LoginSteps.java’:
package stepDefinitions;
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
import io.appium.java_client.MobileElement;
import io.appium.java_client.android.AndroidDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import java.net.URL;
public class LoginSteps {
private AndroidDriver<MobileElement> driver;
@Given(“the user is on the login screen”)
public void the_user_is_on_the_login_screen() throws Exception {
// Set up Appium driver and capabilities
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(“deviceName”, “deviceName”);
capabilities.setCapability(“platformName”, “Android”);
// Other capabilities as needed
URL appiumServerURL = new URL(“http://localhost:4723/wd/hub”);
driver = new AndroidDriver<>(appiumServerURL, capabilities);
// Navigate to the login screen
}
@When(“they enter valid credentials”)
public void they_enter_valid_credentials() {
// Perform actions to enter valid credentials
}
@Then(“they should be logged in”)
public void they_should_be_logged_in() {
// Assertions or verifications for successful login
}
}
Set Up Appium Configuration
Configure the settings required for Appium to interact with your mobile application. This includes specifying the device name, platform version, app path, and any desired capabilities. Desired capabilities define the characteristics of the device and app you want to test. These configurations guide Appium in setting up the testing environment accurately, ensuring that your tests are executed on the correct platform with the appropriate app version.
Implement Test Runner
Design and implement a test runner class responsible for orchestrating the execution of your Cucumber scenarios. This class, located within the `test/java` directory, uses the `@CucumberOptions` annotation to define key parameters. These parameters include the paths to your feature files and step definitions. You can also set up additional options, such as formatting preferences for the generated reports. The test runner acts as the entry point for your test execution and coordinates the integration between Cucumber, Appium, and your test scripts.
for example, ‘TestRunner.java’:
package test;
import org.junit.runner.RunWith;
import io.cucumber.junit.Cucumber;
import io.cucumber.junit.CucumberOptions;
@RunWith(Cucumber.class)
@CucumberOptions(
features = “src/test/resources/features”,
glue = “stepDefinitions”,
plugin = {“pretty”, “html:target/cucumber-reports”}
)
public class TestRunner {
}
Run Tests
Run your automated tests by executing the test runner class. When you initiate the test run, Appium will launch the mobile application on the specified device or emulator and follow the steps defined in your feature files. It interacts with the app based on the logic you’ve written in your step definition methods. The execution process mirrors the flow of the scenarios in your feature files, allowing you to verify the behaviour and functionality of your application through automation.
View Reports
Cucumber provides comprehensive reports that detail the outcomes of your test execution. These reports are generated in formats like HTML, JSON, or XML. You can use these reports to gain insights into the test results, including passed and failed scenarios, along with detailed error messages. Viewing these reports helps you assess the effectiveness of your tests and identify any issues or bugs in the application.
Refine And Iterate
After reviewing the test results, iterate and refine your automation scripts as needed. If any tests fail, investigate the cause by examining the error messages, logs, and screenshots from the test reports. Adjust your step definitions, fix any bugs, or update the feature files to reflect changes in application behaviour or requirements. This iterative process ensures that your automation suite remains up-to-date, reliable, and aligned with the evolving state of the application.
Best Practices For Writing Cucumber Automation Testing Scripts
Writing effective and maintainable Cucumber automation testing scripts requires following best practices that ensure readability, maintainability, and efficient collaboration among team members. So, here are some best practices to consider:
Use Descriptive Feature Files: Feature files should clearly convey what aspect of the application is being tested. Use concise yet descriptive titles and descriptions to communicate the intended behaviour. For example:
Feature: User Registration
As a new user
I want to create an account
So that I can access the application
Precise Scenario Outlines: Scenario Outlines allow parameterization of scenarios. They are especially useful when testing variations of the same behaviour. Clearly define placeholders in the steps and provide example sets for better readability and understanding.
Avoid Technical Details In Feature Files: Feature files are meant to capture the application’s behaviour from a user’s perspective, not its technical implementation. Avoid mentioning UI elements, locators, or low-level technical details in feature files.
Use Hooks Sparingly: Hooks can be used for setup and teardown, but overusing them can lead to complex scenarios and diminished maintainability. Consider alternatives like Background sections for common setups.
Readable Step Definitions: Write step definitions that are easy to read and understand. Use descriptive method names and clear comments to explain the purpose of each step. Avoid abbreviations and overly cryptic names.
Follow The DRY Principle: Reuse step definitions to avoid duplicating code. If similar steps occur across scenarios, create shared methods or context-specific hooks that encapsulate common actions.
Clear And Expressive Gherkin Syntax: Write Gherkin scenarios in a clear and expressive manner. Use simple language that captures the expected behaviour. Avoid ambiguity and complex wording.
Meaningful Tags: Use tags to categorize and group scenarios based on their purpose, such as @smoke, @regression, or custom tags. Tags make it easier to filter and execute specific subsets of tests.
Use Data Tables And Examples: Data tables and examples in Scenario Outlines allow you to test different data inputs or scenarios using the same steps. This enhances test coverage without bloating the feature files.
Version Control: Store your feature files, step definitions, and other testing code in a version control system like Git. This ensures that changes are tracked and multiple team members can collaborate seamlessly.
Regular Review And Refactoring: Periodically review your feature files and step definitions. Eliminate redundant steps, refactor complex logic, and keep the codebase clean. This helps maintain the efficiency of your tests over time.
Error Handling And Assertions: Implement appropriate error-handling techniques and use assertions to validate expected outcomes. Well-crafted assertions with descriptive failure messages aid in debugging failures.
Use Comments Sparingly: While comments can be helpful, strive to write self-explanatory code that doesn’t heavily rely on comments. Use comments only where code complexity requires additional explanation.
Collaboration And Communication: Maintain clear communication among team members regarding changes in feature files, step definitions, and overall testing strategy. Collaboration tools and discussions help align everyone’s efforts.
Conclusion
Integrating Cucumber with Appium brings the best of both worlds – the flexibility of using Appium’s extensive support for automating various types of mobile applications and the simplicity and readability of Cucumber’s Behavior-Driven Development (BDD) approach. By following the steps outlined in this article, you can seamlessly configure Cucumber and Appium to work together, allowing for easy script maintenance and effective communication across your team. This blend offers a robust framework for mobile test automation that is both scalable and understandable, making it a go-to choice for quality assurance teams aiming for high-quality, user-focused mobile applications.