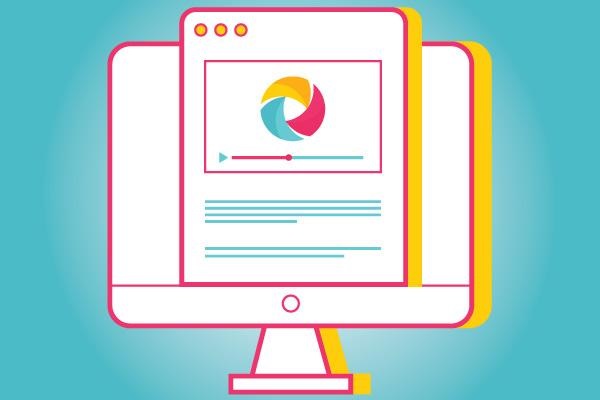
A Beginner’s Guide to Appium JavaScript Testing
Appium is one of the most popular names for initiating automation test cases on mobile applications. Maintaining the compatibility of a modern application on the mobile platform is crucial for expanding the audience base of the company. This is because various surveys prove that more than 53% of the total traffic comes from multiple mobile devices. Some of the most commonly used mobile devices include smartphones, smartwatches, and even tablets. While performing mobile app testing, the app testers have to consider the diversity in this segment and need to implement accurate test instances. Modern application testing processes like Agile methodologies can help companies synchronize the working mechanism of the development and testing teams.
Since JavaScript is one of the most popular programming languages in the modern app development segment, almost all app developers and testers have a basic understanding of this language. So with this article, we are going to guide the new testers through the process of executing Appium test cases while developing the automation test scripts in the JavaScript programming language.
Exploring Automation Testing and Appium
To properly understand the standards of the modern app testing industry, we need to explore deeper into the world of automation testing. This is an efficient way of eliminating the involvement of human testers in the application testing cycle. As a replacement, the system will use a series of predetermined parameters for replicating various forms of human interactions while communicating with the app. Based on these communications, the system will generate a detailed test report showing the usability, stability, and user interactivity of the app undergoing the development process. In this segment, the testers also have the liberty of making significant changes in these data for customizing the reports according to the project requirements.
Considering all the modern standards, the proper implementation of test automation can benefit the app development cycle in the following ways:
- Using test automation, the application developers can execute the test cases on complex apps within a few days. This is because automation testing uses parallel testing to execute thousands of different test instances at the same time.
- Automation testing also improves the quality of the application as it does not consist of errors that can arise during human involvement. It also has a massive role in improving the efficiency of the testing process as it can run the test case in 24 hours around the clock which is beyond human capabilities.
- Automation testing will help boost the company’s resources in the long run. This is because it will help the app-developing companies eliminate the expenses and hassle involved with maintaining a human testing team.
- Automation testing also supports the integration of various tools, platforms, and plugins for further boosting the efficiency of the testing cycles.
We can define Appium as a modern tool for initiating UI test cases on mobile applications. Using the Appium API, the app testers can also verify the functioning of native apps, hybrid mobile apps, and mobile-based web applications. The open-source infrastructure of Appium ensures developers can use all of its features without worrying about any platform fees. The Appium API is also very simple and welcoming to the new automation testers and developers. This tool also allows the application developers to initiate and execute the automation test cases on both Android and iOS applications.
Diversity of the Modern Mobile App Testing Segment
As we already mentioned earlier, the mobile app testing segment is one of the most diverse parts of the app development industry. This is because the mobile platform consists of multiple operating systems like Android, iOS, and Windows. Android is the dominant competitor which has more than 70% of users globally. In the Android segment, more than 1,000 devices come to the market annually. So this data is enough to justify why the app developers cannot generalize the test cases while working with the mobile platform. We can also say that restricting the test cases to emulation software also restricts the compatibility and accuracy of the test cases. Thus real device testing becomes one of the most important components of the mobile app industry.
Using real device testing, the app developers can verify the basic functioning of the app infrastructure based on various physical parameters like unstable bandwidth, change in app demographics, low battery, or user interaction errors.
LambdaTest and its Role in Appium Automation
To eliminate the hassle and expense of a physical device, app developers can integrate cloud-based solutions like LambdaTest. LambdaTest is a highly sought-after cloud-based cross browser testing platform that enables you to carry out automated testing on over 3000 browsers, devices, and operating systems, making it a top choice among other cloud testing platforms. While using this platform for executing Appium test cases, the developers can run the test instances on thousands of different real devices and browser combinations at the same time. This process also helps to improve the accuracy of the test cases as it integrates multiple reports from different devices and software.
While using the LambdaTest real device testing cloud for executing Appium test cases, the developers can utilize the following features:
- The LambdaTest cloud also provides access to legacy devices and older browser versions that are impossible to find in the present market. Using these test instances, the app developers can expand the compatibility of their application to older browser versions which are still used by a significant section of the customer base.
- The LambdaTest real device testing cloud also supports the integration of parallel test execution to initiate thousands of different Appium test cases on different devices, operating systems, and browser versions at the same time.
- While using LambdaTest, the app developers will have access to an elaborate support system consisting of over-the-call support, a mailing feature, and life chat. All of these features ensure that the app developers have adequate information regarding the various tools and features of this platform. They also have access to an open-source community where testers and developers can discuss various issues and features with modern testing cycles.
- Finally, LambdaTest will generate a detailed test report after executing the Appium test cases. These reports will consist of elaborate test logs and visual representations consisting of videos and screenshots. The developers can analyze this data to quickly highlight the faulty element in the core infrastructure of the application. So we can say that LambdaTest has a critical role in simplifying the troubleshooting and debugging process.
Executing Appium JavaScript Test Scripts
By integrating Appium with the LambdaTest real device testing cloud, the app developers can easily execute the JavaScript automation test cases. To assist the new automation testers, we have mentioned all the required steps and prerequisites in the chronological order:
- Prerequisites:
- For newcomers to LambdaTest, the first step is to navigate to the official website and sign up for a new account. After purchasing the license, the app developers will receive the username and access key that will allow them to access the LambdaTest real device testing cloud.
- Now, the application developers have to set up their project by creating a new directory for the Appium testing project. After this, the app testers have to navigate to this project using the terminal window.
- Now, the application developers need to initialize a new Node.JS project by running ‘npm init’. By entering the following code in the terminal window, the application developers will be able to install the required dependencies for executing the test cases.
npm install appium appium-wait-plugin wd axios
- The developers also have to ensure that they have the Java client library installed for both Appium and Selenium.
- For the proper execution of the JavaScript test cases, the application developers also have to download and install Maven from the official website.
- Writing the Appium Test Scripts:
- Now, that the application developers have finished configuring the Appium and LambdaTest environments, it is time for them to write their first Appium test script using the JavaScript programming language. In case the app testers are using existing JavaScript automation test scripts, they must modify the test parameters to ensure that they are compatible with the LambdaTest API.
- Importing the Required Libraries and Setting Up Appium
- To properly execute the Appium test cases using LambdaTest, the app developers have to import the required libraries and set up the Appium environment. For this process, they simply have to enter the following code in the native environment of the terminal window:
const wd = require(“wd”);
const axios = require(“axios”);
const serverConfig = {
protocol: “https”,
host: “hub.lambdatest.com”,
port: 80,
path: “/wd/hub”,
};
const capabilities = {
platform: “Android”, // or “iOS”
deviceName: “Samsung Galaxy S20”, // Specific device name from LambdaTest
browserName: “chrome”, // Use “chrome” for Android
// Other capabilities like platformVersion, app, etc.
};
const driver = wd.promiseChainRemote(serverConfig);
- Configuring the Test Compatibilities
- While working with mobile apps, the app developers need to configure the required capabilities for streamlining the test instances. Some of the most important test capabilities include the required device, operating system, browser version, and other parameters. Using the following code snippet, the application developers can easily configure the test capabilities for the Appium test instances:
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability(“deviceName”, “Galaxy S20”);
capabilities.setCapability(“platformVersion”, “11”);
capabilities.setCapability(“platformName”, “Android”);
capabilities.setCapability(“isRealMobile”, true);
capabilities.setCapability(“app”, “YOUR_APP_URL”); //Enter your app url
capabilities.setCapability(“deviceOrientation”, “PORTRAIT”);
capabilities.setCapability(“build”, “Java Vanilla – Android”);
capabilities.setCapability(“name”, “Sample Test Java”);
capabilities.setCapability(“console”, true);
capabilities.setCapability(“network”, false);
capabilities.setCapability(“visual”, true);
capabilities.setCapability(“devicelog”, true);
- Executing the Appium Test Cases
- Now, it is finally time for the App testers we execute the Appium test cases using the LambdaTest real device testing cloud. For this process, the app developers simply have to enter a specific command depending on the target platform. We have mentioned the required commands below:
Android Command:
mvn test -P android
iOS Command:
mvn test -P ios
- Analyzing the Test Reports:
- After successfully executing the Appium test cases, LambdaTest will automatically display the test reports on the test console. This report will be shown in the command-line interface when the app developers are running the test cases using a terminal or command prompt.
The Final Verdict
Through this article, we aim to guide the new automation testers toward the execution of Appium test cases using the JavaScript programming language. This process is further simplified with the integration of LambdaTest and other modern automation testing tools. We also emphasized the integration of automation testing in the modern application development life cycles. So, it is very important for the developers to constantly update the information and knowledge regarding all the new automation testing trends and innovations. It is also a wise decision to constantly gain more information about the changing requirements of the target audience. All this data will help the app developers to customize their applications for a wider user reach.